Step 1 - Sign up for a Google Maps API key:
- Go to http://code.google.com/apis/maps/ and Sign up for a Google Maps API key -> enter your web site URL in My web site URL, and check I have read and agree with the terms and conditions, then click Generate API Key.
For example: I'll sign up for a Google Maps API key for my website http://skyofflowers.googlepages.com.
The result: - Now, you can copy example code to make an example web page and see how Google Maps works.
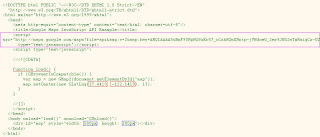
<script src="http://maps.google.com/maps?file=api&v=2&key=ABQ...
type="text/javascript"></script>
This Javascript contains API key "ABQ..." is required for page using Google Maps. Use the API key you've got at Step 1.
function load() {
if (GBrowserIsCompatible()) {
var map = new GMap2(document.getElementById("map"));
map.setCenter(new GLatLng(37.4419, -122.1419), 13);
}
}
This is main function to load a map.
var map = new GMap2(document.getElementById("map")); //create map object
map.setCenter(new GLatLng(37.4419, -122.1419), 13); //set the center view of map ~ where you want to display.
Method setCenter(...) has 2 arguments:
- Latitude and Longtitude as a GLatLng object
- Zoom level
And maybe.... you want to know how to get latitude and longtitude of a place. Go to http://maps.google.com and search for one.
<body onload="load()" onunload="GUnload()">
This HTML script calls load() function defined above. It also has onunload event to prevent memory leaks
<div id="map" style="width: 500px; height: 300px"></div>
This HTML script makes a view to display map on web page. You can customize the width and height of the view.
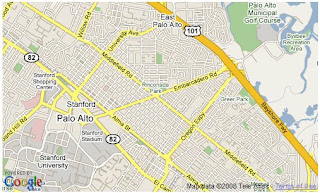
Step 3 - Customize your map:
Based on the example code, you can modify it to suit your need.
1. Customize map type: use method
setMapType(map_type)
. Standard map types:G_NORMAL_MAP
- the default viewG_SATELLITE_MAP
- showing Google Earth satellite imagesG_HYBRID_MAP
- showing a mixture of normal and satellite viewsG_DEFAULT_MAP_TYPES
- an array of these three types, useful for iterative processing
var map = new GMap2(document.getElementById("map_canvas"));
map.setMapType(
G_HYBRID_MAP
); //showing a mixture of normal and satellite views
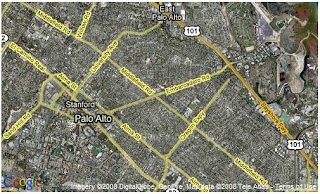
2. Show a welcome message like a floating balloon: use method openInfoWindow(...)
map.openInfoWindow(map.getCenter(), document.createTextNode("Hello, world"));
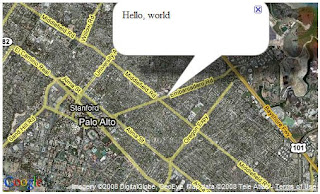
3. Add Large Map control:
map.addControl(new GLargeMapControl());
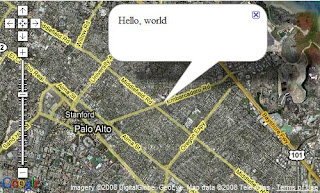
4. Add Small Map control:
map.addControl(new GSmallMapControl());
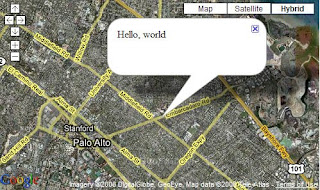
5. Add Zoom In & Zoom Out buttons:
map.addControl(new GSmallZoomControl());
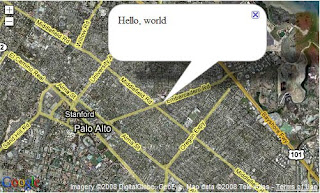
6. Add Map Type control:
map.addControl(new GMapTypeControl());
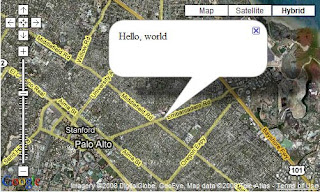
7. Add a marker:
var options = {
title: "Welcome you!",
icon: G_DEFAULT_ICON
};
var marker = new GMarker(new GLatLng(37.4419, -122.1419), options);
map.addOverlay(marker);
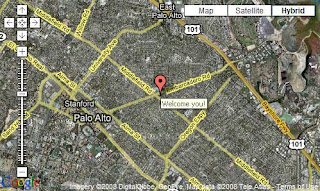
8. Add a marker:
var marker = new GMarker(new GLatLng(37.4419, -122.1419));
GEvent.addListener(marker, "click", function() {
var html = '<div style="width: 210px; padding-right: 10px;"><a href="http://www.blogger.com/signup.html">Welcome you!<\/a><\/div>';
marker.openInfoWindowHtml(html);
});
map.addOverlay(marker);
GEvent.trigger(marker, "click");
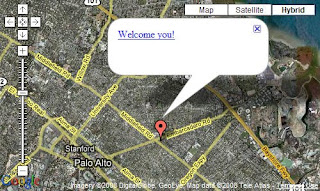
Here is a modified sample.
References:
0 comments:
Post a Comment